[unity] 모듈 제작 : 팝업 시스템 만들기(4)
Popup에 필요한 Buttons 클래스와 Popup의 정보를 가지고 있는 Info 클래스를 작성해보자.
1. 스크립트 작성
1. Popup : 팝업 객체에 붙는 컴포넌트로 팝업 내 UI 요소들을 컨트롤하는 클래스
2. PopupManager : 팝업을 관리하는 매니저 클래스
3. PopupAnimator : 팝업 애니메이션을 관리하는 클래스
3개 클래스는 이전 편을 참고하자.
2022.05.24 - [unity3d/Modules] - [unity] 모듈 제작 : 팝업 시스템 만들기(3)
[unity] 모듈 제작 : 팝업 시스템 만들기(3)
팝업을 관리하는 매니저 클래스인 PopupManager Class를 제작해보자. 1. 스크립트 작성 제작 해야될 스크립트는 총 7가지로 각 클래스 별 기능 설명을 하자면 다음과 같다. 1. Popup : 팝업 객체에 붙는
wonjuri.tistory.com
4. PopupButton : 팝업 전용 버튼 클래스
5. PopupButtonInfo : 팝업 전용 버튼 정보 클래스
6. PopupButtonType : 팝업 버튼 타입 정의 Enum
7. PopupInfo : 팝업 생성을 위한 정보를 담은 클래스
4. PopupButton
- UGUI.Button 을 상속받아 확장 클래스를 만들자.
- 버튼 클릭시 상위의 PopupManager로 클릭 이벤트를 넘겨준다.
using UnityEngine;
using UnityEngine.UI;
namespace Container.Popup
{
public class PopupButton : Button
{
protected PopupManager obj_manager = null;
public PopupButtonType enm_type = PopupButtonType.None;
public PopupButtonType Type
{
get { return enm_type; }
set { enm_type = value; }
}
public string Name
{
get { return GetComponentInChildren<Text>(true).text; }
set { GetComponentInChildren<Text>(true).text = value; }
}
public Color Color
{
get
{
return GetComponent<Image>().color;
}
set
{
GetComponent<Image>().color = value;
}
}
protected override void Start()
{
base.Start();
if (obj_manager == null)
{
obj_manager = this.GetComponentInParent<PopupManager>();
}
}
/// <summary>
/// 버튼 클릭시 매니저로 이벤트를 보내는 함수
/// </summary>
/// <param name="eventData">Event data.</param>
public override void OnPointerClick(UnityEngine.EventSystems.PointerEventData eventData)
{
base.OnPointerClick(eventData);
if (obj_manager != null)
{
obj_manager.OnClosePopup(enm_type);
}
}
}
}
5. PopupButtonInfo
- PopupButton의 정보만을 가지고 있다.
using UnityEngine;
using System;
[Serializable]
public class PopupButtonInfo
{
public string str_name;
public Color color_button;
}
6. PopupButtonType
- Description 속성을 통해 버튼의 텍스트를 설정한다.
using System.ComponentModel;
using System;
namespace Container.Popup
{
/// <summary>
/// Description 이 버튼의 텍스트로 들어감.
/// </summary>
[Serializable]
public enum PopupButtonType
{
None = 0,
[Description("예")]
Yes = 1,
[Description("아니오")]
No = 2,
[Description("확인")]
Confirm = 3,
[Description("닫기")]
Close = 4,
[Description("다시하기")]
RePlay = 5,
[Description("홈으로")]
GoHome = 6,
[Description("게임종료")]
FinishGame = 7,
[Description("시작")]
Start = 8
}
}
7. PopupInfo
- 가장 핵심 클래스이다.
- 빌더 패턴을 사용하여, 팝업의 다양한 객체 정보를 담는다.
- 요소가 많을 수록 해당 패턴을 사용하는 게 유용해진다.
- 팝업 객체를 만들때 필요한 타이틀 정보, 내용 정보, 버튼 정보, 버튼 리스너, 애니메이션, 화면 Pause 여부 등을 설정할 수 있다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
namespace Container.Popup
{
public class PopupInfo
{
private PopupInfo(Builder builder)
{
this.Title = builder.Title;
this.Content = builder.Content;
this.Buttons = builder.Buttons;
this.Listener = builder.Listener;
this.Animation = builder.Animation;
this.PauseScene = builder.PauseScene;
}
public bool PauseScene { get; private set; }
public string Title { get; private set; }
public string Content { get; private set; }
public PopupButtonType[] Buttons { get; private set; }
public System.Action<PopupButtonType> Listener { get; private set; }
public PopupAnimationType Animation { get; private set; }
public class Builder
{
public Builder()
{
this.Title = string.Empty;
this.Content = string.Empty;
this.Buttons = null;
this.Listener = null;
this.Animation = PopupAnimationType.None;
this.PauseScene = false;
}
internal string Title { get; private set; }
internal string Content { get; private set; }
internal bool PauseScene { get; private set; }
internal PopupButtonType[] Buttons { get; private set; }
internal System.Action<PopupButtonType> Listener { get; private set; }
internal PopupAnimationType Animation { get; private set; }
public Builder SetTitle(string title)
{
this.Title = title;
return this;
}
public Builder SetContent(string content)
{
this.Content = content;
return this;
}
public Builder SetButtons(params PopupButtonType[] buttons)
{
this.Buttons = buttons;
return this;
}
public Builder SetListener(System.Action<PopupButtonType> listener)
{
this.Listener = listener;
return this;
}
public Builder SetAnimation(PopupAnimationType animation)
{
this.Animation = animation;
return this;
}
public Builder SetPauseScene(bool isPause)
{
this.PauseScene = isPause;
return this;
}
public PopupInfo Build()
{
return new PopupInfo(this);
}
}
}
}
사용 방법은 다음편에 이어서~
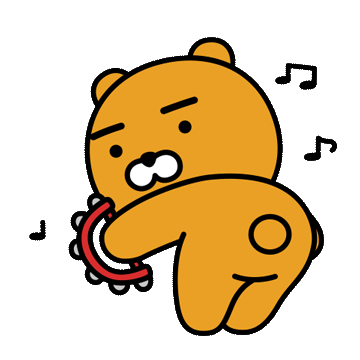