[unity] Open Path Utility editor class
Unity의 UIElements를 사용하여, 자주 사용하는 폴더를 바로 열어볼 수 있는 기능을 가진 Open Path Utility Editor Class를 작성해보자.
1. Open Path Utility Class 제작 이유
- 유니티를 하다 보면 항상 특정 폴더를 열어야 할 때(Asset Path, PersistentDataPath, StreamingAssetPath 등) 파인더를 열어서, 해당 폴더를 찾아가곤 했다. 개발할 때 이런 시간적 비용이 점점 커짐에 따라, 해당 기능을 유니티 에디터 기능으로 만들고, 유니티에서 바로 폴더를 열 수 있는 기능을 제작해 놓으면, 상당 부분 개발 시간(비용)을 줄일 수 있다.
2. Unity UIElements 소개
- UIElement는 unity 2019버전부터 새롭게 선보인 UI 시스템이다.
- 기존 UGUI 시스템과 다르게 웹 기술에 기반하여 구현 및 동작하도록 구성되어 있다(xml, css, jQuery 등)
- 개인적으로 기존 웹 기반 기술을 가진 개발자라면 접근이 쉬울 것으로 생각되지만, 앱 위주 개발을 했던 개발자라면 조금 어려울 수 있을 것 같기도 하다.
Runtime dev UI | Runtime game UI | Editor | 장단점 | |
IMGUI | 디버깅용 | 권장하지 않음 | 가능 | 에디터에서 적용하기 간편함 |
UGUI | 가능 | 가능 | 불가능 | 대부분 게임UI에 많이 사용 중, 사용성 편리함 |
UIElements | 2019.x 부터 가능 | 2020.x 부터 가능 | 2019.1 부터 가능 | 에디터 및 런타임 게임 UI에 동시 사용 가능 적용 방법 어려움 |
3. Unity UIElement 생성
- Assets 폴더 적당한 곳에 Utils 폴더를 만들고 Editor 폴더를 만들어 준다.
- 빈 공간에서 마우스 우클릭 -> Create -> UIElements -> Editor Window로 생성한다.
- OpenPathUtility 라는 이름으로 생성한다.
- 생성을 하게 되면 총 3개의 파일이 생성된다.
.cs 파일 : Editor Window 클래스
.uss 파일 : 디자인(스타일) 정보를 가지고 있는 파일
.uxml 파일 : UI 위치 정보 및 화면의 정보를 가지고 있는 xml 파일
4. Elements Editor Window 생성
- .uxml 파일을 열고 기존 내용을 삭제하고, 버튼을 생성해주는 아래 코드를 넣어준다.
<ui:UXML xmlns:ui="UnityEngine.UIElements" xmlns:uie="UnityEditor.UIElements">
<ui:Button name="dataPath" text="Application.dataPath" />
<ui:Button name="persistentDataPath" text="Application.persistentDataPath" />
<ui:Button name="streamingAssetsPath" text="Application.streamingAssetsPath" />
<ui:Button name="temporaryCachePath" text="Application.temporaryCachePath" />
</ui:UXML>
- .cs 파일을 열고 MenuItem 부분의 경로를 "Utils/OpenPathUtility"로 수정해준다.
- CreateGUI() 함수의 label 항목을 삭제해 준다.
- 코드는 아래를 참고하자.
using UnityEditor;
using UnityEngine;
using UnityEngine.UIElements;
using UnityEditor.UIElements;
public class OpenPathUtility : EditorWindow
{
[MenuItem("Utils/OpenPathUtility")]
public static void ShowExample()
{
OpenPathUtility wnd = GetWindow<OpenPathUtility>();
wnd.titleContent = new GUIContent("OpenPathUtility");
}
public void CreateGUI()
{
// Each editor window contains a root VisualElement object
VisualElement root = rootVisualElement;
// Import UXML
var visualTree = AssetDatabase.LoadAssetAtPath<VisualTreeAsset>("Assets/Modules/Utils/OpenFolder/Editor/OpenPathUtility.uxml");
VisualElement labelFromUXML = visualTree.CloneTree();
root.Add(labelFromUXML);
}
}
- 저장 후 보면 상단에 Utils 라는 Editor Menu가 생기고, 해당 메뉴를 클릭하면 OpenPathUtility EditorWindow가 생성되는 모습을 볼 수 있다.
5. 버튼 이벤트 스크립트 작성
- Editor Window의 모습이 완성되었으니, 각 버튼을 클릭했을 때 해당 폴더가 열리는 기능을 작성해보자.
- .cs 스크립트를 열고 CreateGUI() 함수에 아래와 같이 스크립트를 추가 작성한다.
public void CreateGUI()
{
// Each editor window contains a root VisualElement object
VisualElement root = rootVisualElement;
// Import UXML
var visualTree = AssetDatabase.LoadAssetAtPath<VisualTreeAsset>("Assets/Modules/Utils/OpenFolder/Editor/OpenPathUtility.uxml");
VisualElement labelFromUXML = visualTree.CloneTree();
root.Add(labelFromUXML);
root.Query<Button>(nameof(Application.dataPath)).First().clickable.clicked += () =>
{
Debug.Log(Application.dataPath);
EditorUtility.RevealInFinder(Application.dataPath);
};
root.Query<Button>(nameof(Application.persistentDataPath)).First().clickable.clicked += () =>
{
Debug.Log(Application.persistentDataPath);
EditorUtility.RevealInFinder(Application.persistentDataPath);
};
root.Query<Button>(nameof(Application.streamingAssetsPath)).First().clickable.clicked += () =>
{
Debug.Log(Application.streamingAssetsPath);
EditorUtility.RevealInFinder(Application.streamingAssetsPath);
};
root.Query<Button>(nameof(Application.temporaryCachePath)).First().clickable.clicked += () =>
{
Debug.Log(Application.temporaryCachePath);
EditorUtility.RevealInFinder(Application.temporaryCachePath);
};
}
- 컴파일 후 다시 Menu -> Utils -> OpenPathUtility를 실행하여 EditorWindow에서 각 버튼을 클릭해 보면. 해당 폴더가 잘 열리는 걸 확인해 볼 수 있다.
5. 응용
- 위 기능을 이용하여, .uxml 파일에 원하는 버튼을 만든 후 cs파일에서 내가 원하는 폴더 경로를 연결해주면 내가 원하는 폴더를 에디터 스크립트를 통해 바로 열어 볼 수 있게 된다.
- Assets 폴더 밑에 Build 폴더를 만든 후, 해당 폴더 바로 열기를 추가한다면 스크립트는 다음과 같다.
- .uxml 에 해당 내용 추가
<ui:Button name="BuildPath" text="BuildPath" />
- .cs CreateGUI() 함수에 해당 내용 추가
root.Query<Button>("BuildPath").First().clickable.clicked += () =>
{
string path = Path.Combine(Application.dataPath, "Build");
EditorUtility.RevealInFinder(path);
};
- 최종 EditorWindow 모습은 다음과 같다.
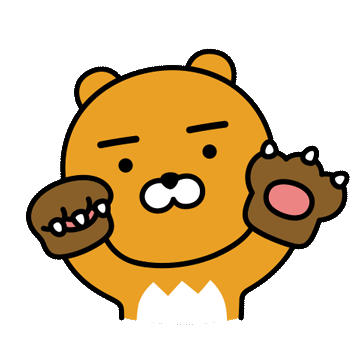
댓글