[unity] 모듈 제작 : 사운드 플레이어 만들기(2)
사운드 플레이어 모듈의 핵심 스크립트인 SoundObject 클래스를 구현해보고, 적용 방법에 대해 알아보자.
1. 사운드 오브젝트 클래스 정의
- 해당 스크립트가 적용되는 GameObject는 AudioSource 컴포넌트를 필요로 한다.
- 사운드가 재생되는 오브젝트에는 SoundObject 컴포넌트가 붙어야 한다.
- 사운드 재생 기능을 구현한다.
- 사운드의 일시정지/해제 기능을 구현한다.
- 코루틴을 사용하여 일정 시간 Delay 후 재생 가능하도록 구현한다.
- 재생이 완료 된 사운드 오브젝트를 Destroy 한다.
2. 사운드 오브젝트 클래스 구현
- Scripts 폴더에 SoundObject.cs 클래스를 생성한다.
- SoundObject.cs 의 코드를 아래와 같이 작성해 보자.
using System;
using System.Collections;
using UnityEngine;
namespace Container.Sound
{
[RequireComponent(typeof(AudioSource))]
public class SoundObject : MonoBehaviour
{
[SerializeField]
private AudioSource audio_Source = null;
private AudioClip audio_Clip = null;
private IEnumerator obj_CurrentRotine = null;
private System.Action action_FinishListener = null;
private bool isStopEnable = true;
private bool isPlaying = false;
[SerializeField]
private bool isDestroyWhenPlayEnd = true;
public AudioSource Audio{
get { return audio_Source; }
set { audio_Source = value; }
}
public AudioClip Clip
{
get { return audio_Clip; }
set { audio_Clip = value; }
}
public bool IsPlaying
{
get { return isPlaying; }
}
private void Awake()
{
if(GetComponent<AudioSource>())
audio_Source = GetComponent<AudioSource>();
}
public void Play(float _volume, float _delaySeconds, bool _isLoop, bool _isStopEnable, System.Action finishLisener = null)
{
if(audio_Source == null)
{
Debug.Log("Audio Source is Null");
return;
}
isStopEnable = _isStopEnable;
audio_Source.clip = Clip;
audio_Source.volume = _volume;
audio_Source.loop = _isLoop;
action_FinishListener = finishLisener;
obj_CurrentRotine = CoPlaySound(_delaySeconds);
StartCoroutine(obj_CurrentRotine);
}
public void Pause()
{
audio_Source.Pause();
}
public void unPause()
{
audio_Source.UnPause();
}
public void Stop()
{
if (isStopEnable)
{
audio_Source.Stop();
if(obj_CurrentRotine !=null)
StopCoroutine(obj_CurrentRotine);
isPlaying = false;
DestroySound();
}
}
IEnumerator CoPlaySound(float delayTime)
{
isPlaying = true;
yield return new WaitForSeconds(delayTime);
audio_Source.Play();
yield return new WaitForSeconds(Clip.length);
isPlaying = false;
if (action_FinishListener != null)
action_FinishListener();
if(!audio_Source.loop)
DestroySound();
}
private void DestroySound()
{
try
{
if(isDestroyWhenPlayEnd)
Destroy(gameObject);
}
catch(Exception e)
{
Debug.Log(e.ToString());
}
}
}
}
- SoundPlayer클래스로 부터 SoundObject 클래스의 Play()함수가 호출된다.
- isStopEnable 멤버 변수를 가지고 있으며, 사운드 재생 중간에 Stop(삭제) 여부를 설정한다.
(UI에서 버튼을 눌러서 생기는 버튼 이펙트 음 같은 경우는 중간에 Stop이 되면 짤리는 것처럼 재생되므로 이런 경우 해당 옵션을 true 로 설정하는게 좋다)
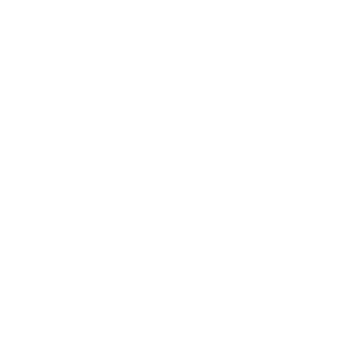
댓글